Use these interview questions and answers to prepare for the technical rounds of your Software Engineering interview.
- For more data structures & algorithms focused questions, check out the post about what’s frequently asked in coding interviews.
- Find behavioral questions and answers in another article.
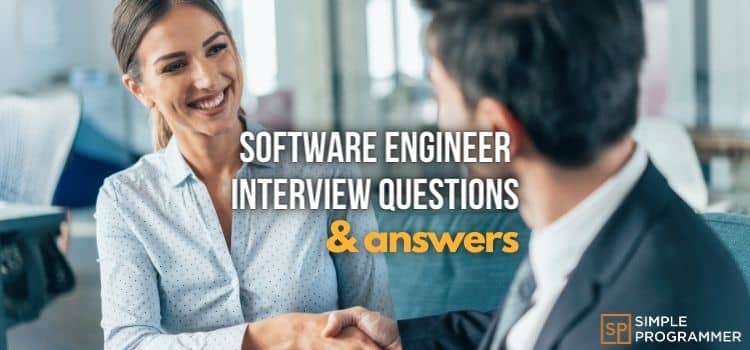
Let’s get to the questions. Good luck in your upcoming interview.
1. Describe your process for creating a software program.
- Understanding the requirements of the software application comes first.
- Then I create a software flowchart detailing how the program will function.
- For each operation within the program, I then write out the code.
- Once I’m finished, I send off the application to QA. An interface can only
- Finally, the end user has to be satisfied with the product. That’s when I know I’ve successfully completed all steps of the process.
2. Please explain Big O notation.
In computer programming, Big O notation is used to measure the runtime of an algorithm. With Big O notation we can compare the efficiency of different solutions to a programming problem.
It shows how an algorithm scales, depending on the size of the input.
3. How do you test for and find bugs?
I’m using the Agile/Waterfall methodologies when developing my software – which means I’m testing my programs at different points, to be able to get ahead of any bugs as early as I can. For this, I’m working with several debugging tools (…).
Additionally, I intermittently have my team review my work. This way I make sure my code is as error-free as possible before I have QA do the last round of tests, and there’s minimal debugs for them to still perform at the end.
4. What is a stack? And which two operations does it perform?
A stack is a data structure. Its unique properties are that it’s using last, in, first out (LIFO) to organize the data – as opposed to a queue, which is FIFO (first in, first out).
The operations of a stack are push and pop. Push is putting the data into the stack at the top. Pop is removing the last piece of data added to the stack.
5. How would you explain cloud computing to a non-technical person?
Most simply put, the cloud is a storage device located remotely. It works like your hard drive, only that you access it over the internet, not on your personal computer.
Programs can also be hosted and run remotely in the cloud, similar to how they do on your PC or smartphone. Today, anytime you do a search or message someone online, or buy from an online store, you’re using the cloud.
6. What is a module? What is modular programming?
A module is an independent block of code that can be called on in the same way a method can. Modules can be shared for use in other systems or by other developers.
The modular programming paradigm states that you should strive to have your software be made up of modules, because they make your code easier to understand and work with. Each program function gets its own module, and everything needed to execute that function is contained in the module.
7. What is a load balancer, and how does it work?
A load balancer routes client requests towards multiple servers, so the load is shared between them, and the request is handled most efficiently and reliably.
Load balancers work by employing different algorithms for distributing the load – such as a Round Robin algorithm, Least Connections algorithm, or Least Time algorithm.
8. In your opinion, what are the skills need to be a successful Software Engineer?
- Problem-solving ability, with regards to projects, teamwork, and the software itself.
- Technical skills, such as mastery of programming principles, software design (using OOP) and coding capabilities (in multiple relevant languages, preferably), as well as testing and debugging skills.
- Interpersonal and communication skills.
- Organizational, planning and leadership/management skills.
9. What is software scope, and what does the process involve?
Software scope defines all actions needed to create the finished software product, as well as what the software will and won’t be able to do. It outlines what will be included in the process, and what won’t be included.
The way to determine software scope is to first define the goals of the project (in light of constraints like the client’s budget), then determine the expected output, spell out each task to be performed, and also clearly define which tasks won’t be done & which features won’t be delivered as part of the project – to keep its scope in check.
10. Can you explain functional vs object-oriented programming?
Object-oriented programming means creating software using classes and objects. Classes specify the variable types and methods for an object. The benefits of using OOP are saving time, making your code more readable, and easier to reuse, debug or be worked on by others.
Functional programming, on the other hand, is based on pure functions – simple, independent blocks of code that take an input, perform a process, and give an output. A pure function’s output only changes if the input is changed, but not with any other impact from the outside.
11. What are the differences between Arrays and Linked Lists?
Method of storing elements. An Array stores data in contiguous memory, whereas a Linked List stores data in a series of nodes, along with a reference (or pointer) to the next node.
Ease of implementation. Arrays are easier to implement than Linked Lists, because with Linked Lists you need to know about dynamic memory allocation and how to manipulate pointers.
Fixed size vs size allocated at runtime. As soon as you declare an Array, the memory needed is allocated to it. With Linked Lists the size is allocated as elements are added to it at runtime.
Speed of memory access. It takes longer to access an element in a Linked List because it could be stored anywhere in the memory zone, which has to then be traversed sequentially.
Efficient use of memory. Memory is utilized more efficiently with a Linked List, because its size isn’t fixed (there can be a lot of unused slots in an Array) – it’s only as large as needed. It’s also possible to use shared memory with Linked Lists.
Speed of adding/removing elements. It’s faster to add or remove an element to/from a Linked List than an Array. An Array has to be completely reindexed when something is added to it or removed from it.
12. What are verification and validation? What’s the difference?
We use verification and validation to check whether the software we’re creating is to specification, up to standard, and does what it’s supposed to do.
When we verify, we statically test the software – without running it – by inspecting and reviewing its documents and code.
When we validate, we dynamically test the software, by running it after it has been compiled. We then look at whether the program executes all its functions, as well as how it performs and how much processing power and memory it uses.
In both cases we compare the results to the requirements established at the outset.
13. What are steps of the Waterfall method of Software Development?
- Requirements
- Design
- Implementation
- Verification
- Maintenance
14. What is a recursive function?
A recursive function is a function that calls itself (directly, or indirectly, using a second function) – until the desired result is achieved. Every time it calls itself, one or more values are changed. The recursion is stopped when the pre-defined base case is reached, the point at which the solution has been reached by running the function multiple times and no more recursion has to be performed.
15. Tell me about monolithic application architecture vs microservices applications architecture.
A monolithic application is created as a cohesive unit, while microservices architecture consists of a number of independent, smaller services.
16. Please define black box testing & white box testing and their differences.
Black box testing is when you test a program without knowing or taking into account its design, structure and code. It only concerns itself with the outward behavior of the software and how it reacts to inputs & outputs.
White box testing, on the other hand, examines the structure and code of the software. It is used in Unit Testing during development, as well as when running Integration Tests.
While black box testing is mostly done by Software Testers/QA, the Software Developer/Engineer uses white box testing during the development phase. In comparison, white box testing is harder to execute and takes more time.
17. Please describe interfaces and abstract classes, and their differences.
Interfaces describe what an object can do – it can be thought of as a contract that defines the properties of an object. It defines methods that any class(es) implementing the interface have to implement. One or multiple (concrete) classes can implement the interface.
Both interface and abstract classes provide data abstraction, which means that unimportant information is hidden from the user. Neither can be instantiated directly – they must be inherited by a concrete class.
Abstract classes are a base for other (sub-)classes. They’re used when you want to have multiple derived classes share a definition.
If objects are similar to each other, it makes sense to use abstract classes. If objects are not related to each other, interface should be used. Because a class can only inherit from one abstract class, but from multiple interfaces, it makes sense to use an interface for when you want a class to behave in a multitude of ways.
18. What are coupling and cohesion, and what is the difference?
Cohesion and coupling are two important ways to ensure proper software design.
Coupling measures the amount of dependency between different modules within the software. Low coupling signifies independence of modules from one another. High coupling refers to a strong interconnection of modules – a change in one module will influence other modules to a large degree.
Cohesion measures the connectedness of parts within each module. High cohesion stands for individual parts working together towards the same outcome. Low cohesion stands for parts of a module differing from each other and serving separate outcomes.
A Software Engineer should aim to create software that has both low coupling and high cohesion, to make it more efficient, readable, and maintainable, as well as less error-prone.
19. Tell me how you come up with estimates for a project.
When estimating a software engineering project, I make sure to take into account the phases of the Software Development Life Cycle (SDLC).
Here’s how I come up with the best estimate for designing, creating, and launching a new software:
- Write out the SDLC for the project to have a high-level overview of what will need to be done.
- Check the requirements for my project (while making sure they’re in line with how my customer has defined his)
- Break down all tasks and deliverables in detail.
- Assign hours to each task, taking into account the team’s abilities. Put limits to each task – if a single task is estimated to be over the limit, split it up into several smaller ones.
20. How would you go about writing your own database server?
- I would first create a read-execute-print loop (REPL).
- Then I’d build an SQL compiler.
- Now I’d use a data structure (like a B-tree) to store the data
- I would write tests for my new database using a TDD tool
- Next I’d add persistence to the database (allowing it to write files to disk)
21. Please explain the concept of inheritance.
Inheritance is one of the main principles of object-oriented programming (OOP). With inheritance, a Software Engineer can set up class hierarchies, with classes/objects (sub or child class/object) inheriting properties and behaviors from a higher-up class (parent or super class).
The benefit of inheritance is cleaner, more efficient, and reusable code that’s less likely to contain errors.
22. Please name some methods you’d use for software protection.
I’d make sure to analyze & communicate security risks up front, and then incorporate principles of secure software development into every step of the Software Development Life Cycle of the application – as well as implementing security testing each step of the way.
It’s important to protect the code not only after, but also during development. This includes safe code storage and employing version control. Frequent code reviews should be performed to check for new vulnerabilities. I would use static code analysis tools to help spot insecurities.
I’ll employ best practices such as:
- Encryption
- Password hashing
- Parameterized SQL queries
- Preventing cross-site scripting
- Executable space protection
- Disallowing unvalidated redirects & forwards
- Error monitoring & exception handling
- Least Privilege principles
- Penetration testing
Additional reading for your Software Engineering interview
For the non-technical, culture fit or personal part of the Software Engineering Interview, check out the post Behavioral Software Engineer Interview Questions.